New useful features in Robot Framework 4 for automation developers
Robot Framework 4 introduces useful new features for automation developers: native IF / ELSE IF / ELSE conditional execution, nested control structures, skipping tasks on condition, and more!
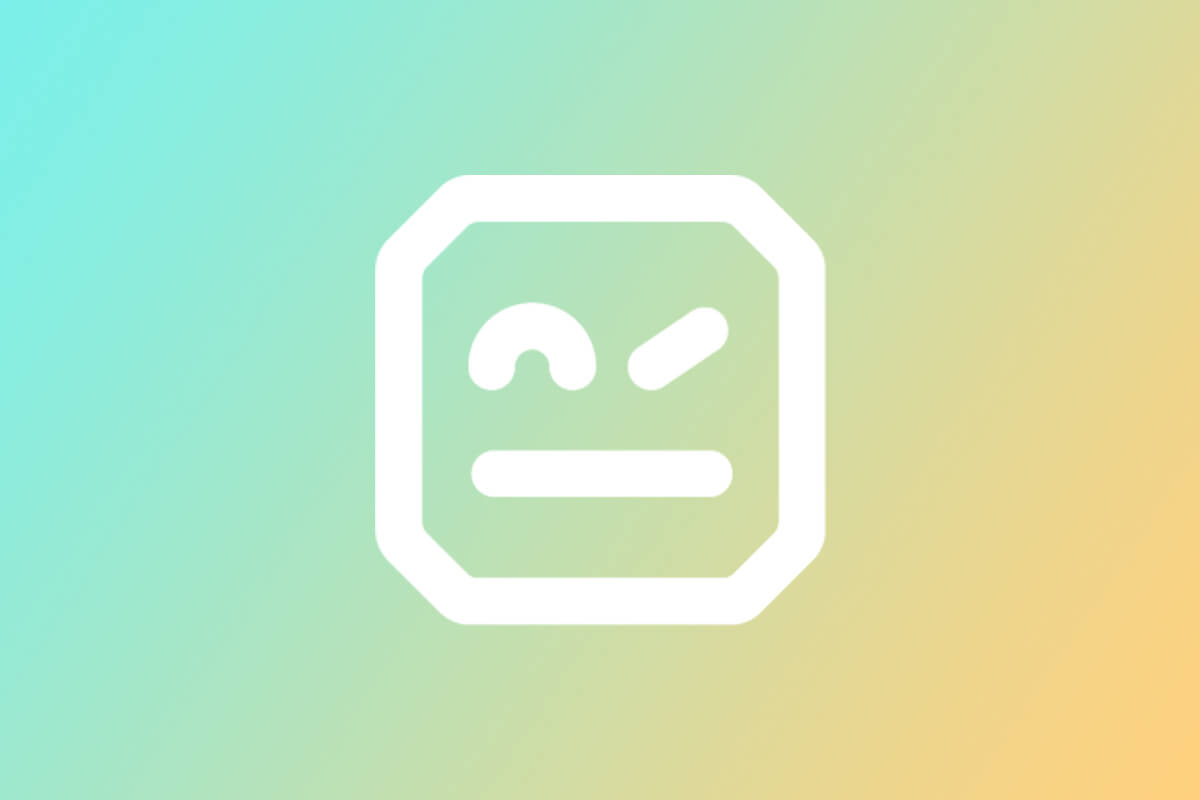
Robot Framework 4
Robot Framework 4 introduces useful new features for automation developers: native IF / ELSE IF / ELSE conditional execution, nested control structures, skipping tasks on condition, and more!
Native IF / ELSE IF / ELSE conditional execution
The simplest processes to automate are usually those where you go from point A to point B without making any decisions on the way.
However, there are times when you might or might not want to do a particular thing based on some condition. For example, if a specific input file already exists, you can skip creating it or retrieving it from some data source.
Robot Framework and Python have language constructs for modeling those decision points.
Robot Framework 3 provided a way to do conditional IF / ELSE execution using the Run Keyword Unless and Run Keyword If keywords:
*** Settings *** Documentation Robot Framework 3 IF / ELSE. *** Variables *** ${CONDITION}= True *** Keywords *** Do conditional execution Run Keyword If ${CONDITION} Log True! Run Keyword Unless ${CONDITION} Log Not True! *** Tasks *** Do IF / ELSE logic Do conditional execution
Robot Framework 4 introduces native IF, ELSE IF, ELSE constructs to achieve conditional execution in your robot, giving you new possibilities for implementing logic branching:
*** Settings *** Documentation Robot Framework 4 IF / ELSE IF / ELSE. *** Variables *** ${CONDITION}= True *** Keywords *** Do conditional execution IF ${CONDITION} Log True! ELSE IF "cat" == "dog" Log Dog! ELSE Log Not True! END *** Tasks *** Do IF / ELSE IF / ELSE logic Do conditional execution
Read Conditional IF / ELSE IF / ELSE execution in Robot Framework for more information on conditional execution in Robot Framework!
Nested control structures
Robot Framework 3 did not natively support nested control structures (such as a FOR loop inside a FOR loop). Robot Framework 4 natively supports nested control structures, such as FOR loops and IF constructs:
*** Tasks *** Use nested control structures FOR ${i} IN RANGE 10 FOR ${j} IN RANGE 10 IF ${j} == ${5} Log Five! END END END
Read How to use for loops in Robot Framework for more information on using loops in Robot Framework!
Skip task execution on condition
Robot Framework 3 supported two different statuses for a task: PASS, or FAIL. Robot Framework 4 introduces a third status: SKIP.
The new SKIP status is helpful in communicating that a specific action (task) was skipped when a particular condition was met:
Documentation How to skip task execution on condition ... using the new skip functionality ... in Robot Framework 4. Library RPA.FileSystem *** Variables *** ${FILE_PATH}= ${CURDIR}${/}file.txt *** Tasks *** Conditional task: Skip if a specific file exists ${file_exists}= Does File Exist ${FILE_PATH} Skip If ${file_exists} Create File ${FILE_PATH} Content *** Tasks *** Main task ${content}= Read File ${FILE_PATH}
Read Conditional IF / ELSE IF / ELSE execution in Robot Framework – Skip task execution on condition: Skip If, Skip for more information on how to use the new skip functionality in Robot Framework 4!
Further reading
Robot Framework has an easy-to-read syntax that you will quickly grasp. You can learn more about Robot Framework from these articles: