Targeting dropdown elements in web applications
How to automate dropdown elements in webpages.
When automating web-based applications, software robot developers often have to target dropdown form elements.
Dropdowns are a widespread interface pattern used for navigation menus, language selectors, and as components in more complicated interface elements. The strategies to automate them depend on how they are built code-wise. The HTML language offers a very simple implementation of a dropdown: the select
tag.
Using a select
tag is the simplest and most accessible way to build a dropdown element. However, it is hard to style the same way in all browsers. Its functionality is pretty basic, so many modern web applications create their own dropdown components with more complicated markup and add interactivity to them using custom JavaScript.
Automating regular select
HTML elements
Here is an example of a dropdown based on a simple select
HTML tag: (you can play with it!)
The example is also available at this URL: https://ccxuf.csb.app
Let's see how a robot that selects the Wall-e
option out of that list would look like. To work on an element in our automation script, we will need to come up with a locator for it.
Read How to find user interface elements using locators in web applications to learn all about locators in web applications.
Using the web developer tools, we can see that the markup for the select item looks like this:
Pretty simple! You have a <select>
HTML tag that wraps a series of <option>
tags.
In this case, the web developers gave us an easy way to locate this element: we can use the id
attribute of the select
tag, which has the value famous-robots
. So, id:famous-robots
will be our CSS based locator.
Setup your environment and create a new robot.
rpaframework
22.0.0
or later is required! Update yourconda.yaml
configuration file.
Selenium: RPA.Browser.Selenium
Add this robot code to the tasks.robot
file:
Robot script explained
In the *** Settings ***
section, we have added a brief description of the robot, and the RPA.Browser.Selenium
library, which gives us keywords to work with web applications, including dropdown menus!
In the *** Tasks ***
section, we have added Select value from dropdown menu
task, which contains our keywords:
Open Available Browser https://ccxuf.csb.app/
opens a new browser and points it to our test page.Wait Until Element Is Visible id:famous-robots
makes sure that the element we want to work with is visible on the page, and we pass it our locatorid:famous-robots
.Select From List By Value id:famous-robots wall-e
will select out of the availableoptions
in the dropdown menu thewall-e
option. TheRPA.Browser.Selenium
library also providesSelect From List By Label
, to which we would have providedWall-e
(capital W) as an argument, andSelect From List By Index
, to which we would have had to send1
, because it starts counting from0
, andWall-e
is the second option in the list.Capture Page Screenshot
: To prove that it worked, we are telling the robot to take a screenshot of the page.[Teardown] Close All Browsers
: We don't need the browser anymore, so let's close it.
Let's run the robot:
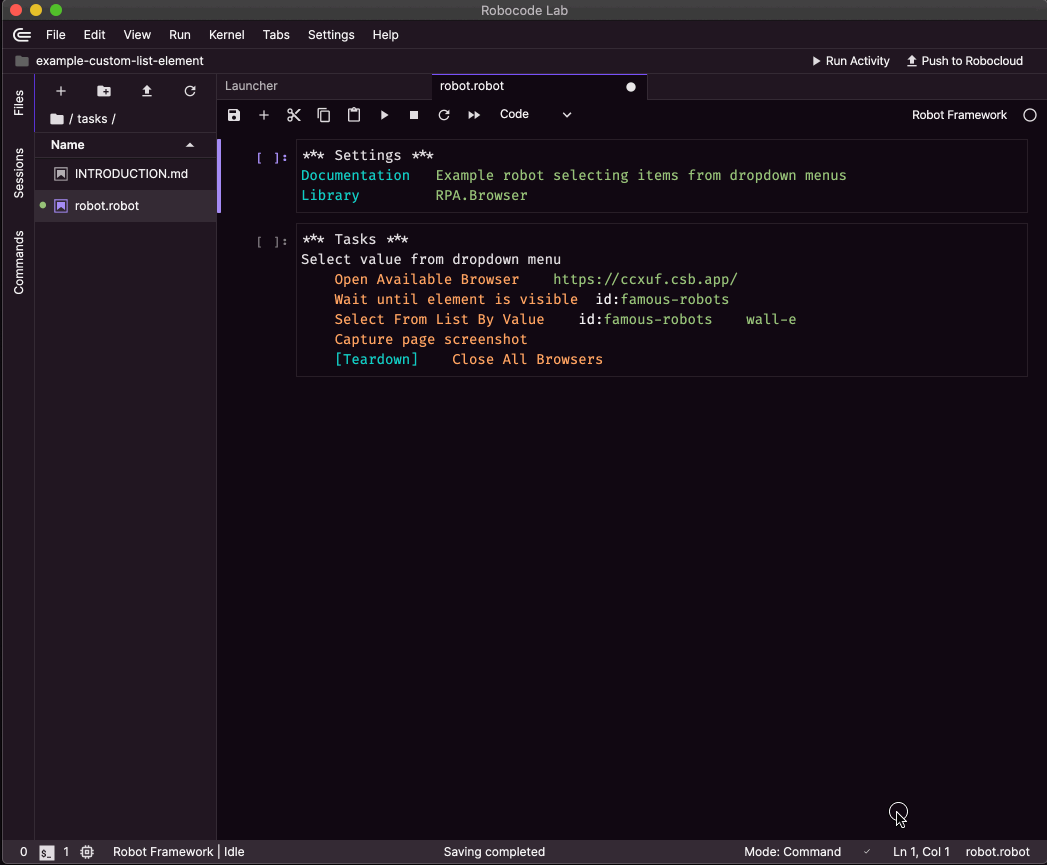
Playwright: Browser
The Playwright-based Robot Framework Browser can also handle HTML select
elements:
Note that there is no need to wait for the element or close the browser, as the library handles those automatically!
Automating custom JavaScript dropdown elements
Here is an example of a custom dropdown based on JavaScript:
The example is also available at this URL: https://e61lk.csb.app/
Let's look at the HTML source of the custom dropdown component, when it is open:
Much more markup this time!
Our robot will need to click on the dropdown to open it, then click on the Wall-e
option from the list that appears.
So, we will need to locate two HTML elements:
- the element that opens the dropdown, which is a
<button>
element with anid
attribute with the valuemy-select
. So our selector will beid:my-select
- the element corresponding to the
Wall-e
value, which is a<button>
element with anid
attribute with the valuedownshift-1-item-1
. So our selector for it will beid:downshift-1-item-1
Selenium: RPA.Browser.Selenium
Let's modify the .robot
code like this:
Robot script explained
In the *** Tasks
section, we have added one task Select value from custom dropdown menu
, which contains our keywords:
Open Available Browser https://e61lk.csb.app/
opens a new browser and points it to our test page containing the custom dropdown element.Click Element When Visible id:my-select
waits for and clicks the button that opens the dropdown menu.Click Element When Visible downshift-1-item-1
will select theWall-e
option.Capture Page Screenshot
: To prove that it worked, we are telling the robot to take a screenshot of the page.[Teardown] Close All Browsers
: We don't need the browser anymore, so let's close it.
Let's run the updated robot:
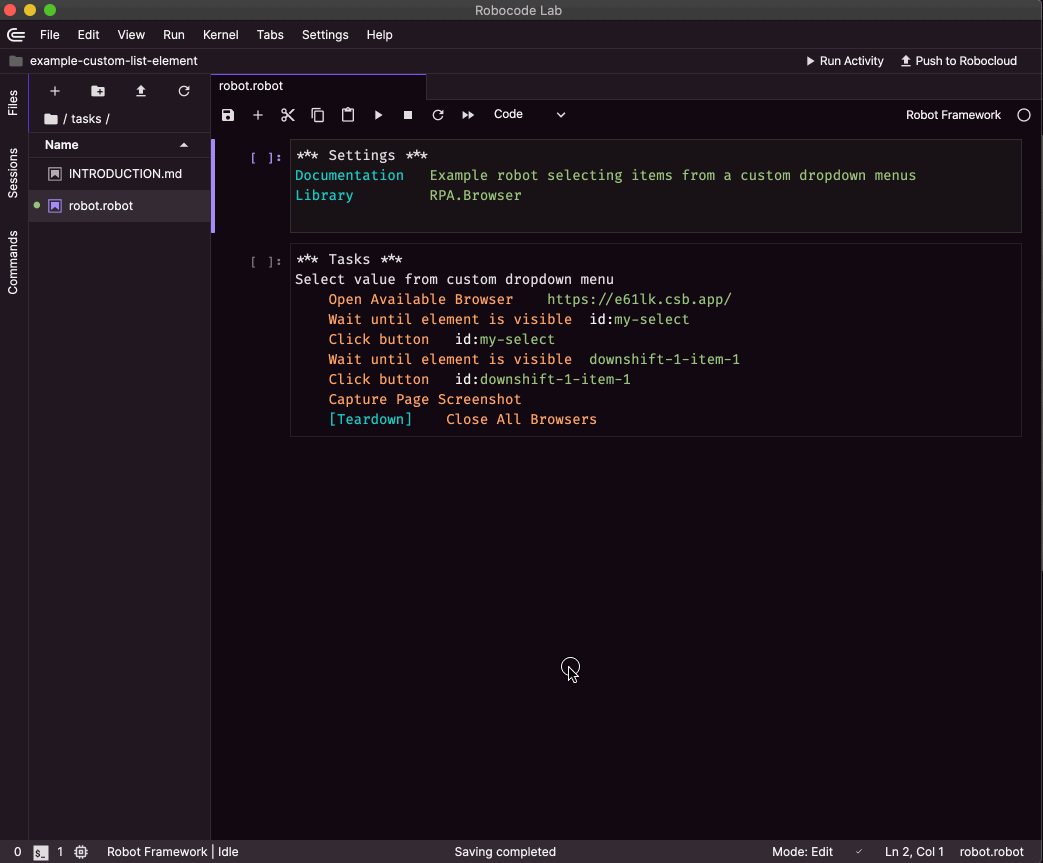
Playwright: Browser
Summary
Automating dropdown menus depends on how they have been built. If they are simple select
HTML elements, you can use the Select From ...
keywords provided by the RPA.Browser.Selenium
library.
Often modern web applications use custom dropdowns that have custom HTML markup. In that case, you have to look at the source, identify the elements that make up the custom component, and target them individually.
Technical information
Created
5 May 2022