Logging into the intranet
Now that it has opened the browser, our robot needs to log into the intranet, using Maria's credentials.
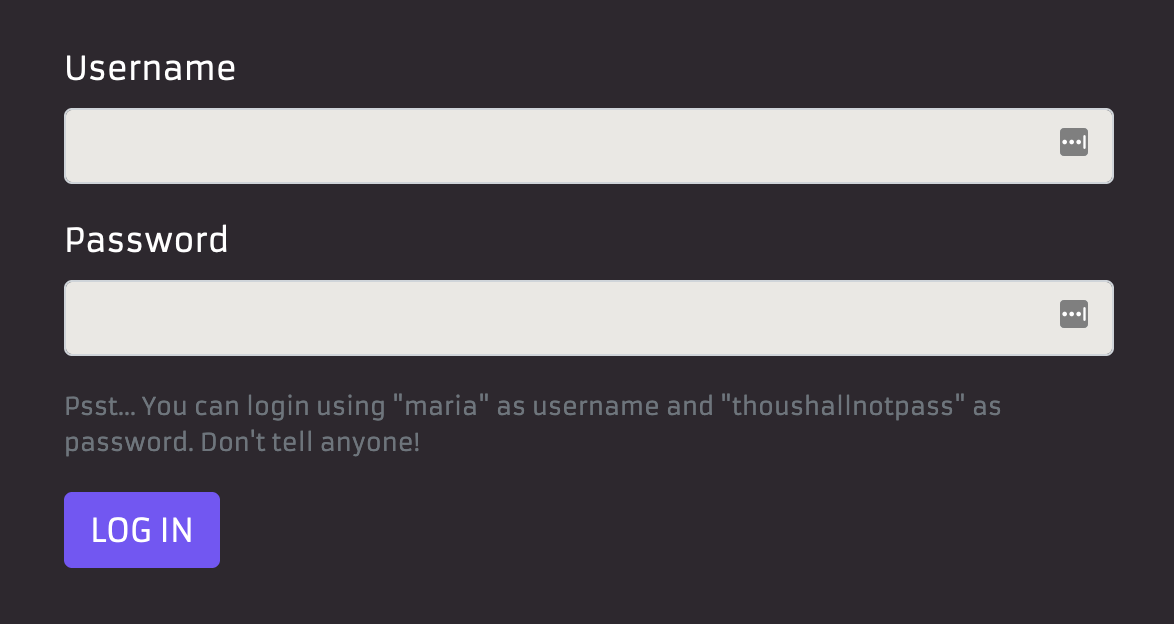
Let's add the next logical step to our task.
We'll call it log_in()
. The @task
function should look like this after adding the step:
Define your new function log_in()
after the definition of open_the_intranet_website()
.
Let's explain what this function does using docstrings:
Ok! Now we have to tell our robot to find the username and the password fields on the page and fill them.
Finding a form field
You, a human being (just assuming!), can see and find things such as forms and fields on a web page. Your robot, however, lacks both vision and the ability to think. It needs precise instructions to find anything.
A web page is written in HTML (Hypertext Markup Language). It defines the meaning and structure of web content. The RobotSpareBin intranet is also built using HTML.
To find forms, fields, and other elements from an HTML web page, your robot needs to know their selectors. Think of a selector as the street address for an element, such as the username field on the RobotSpareBin intranet login page. These selectors along with their name and other instructions are stored in locators, in a file called locators.json
, from which they can be referenced to in the code or used directly as in-line selectors. Don't worry, this will start to make more sense when we start using the locators.
You don't have to inspect the HTML elements during this course unless you want to. All the HTML snippets are provided right here for your convenience. Still, learning how to find and interact with elements is the bread and butter of web automation, so make some time to learn it well eventually!
Inspecting elements in Chrome looks something like this (all the major browsers provide similar tools):
This is what the username field HTML markup looks like in the intranet login form:
<input type="text" id="username" name="username" required="" class="form-control"/>
It is an input
element. It has several attributes
, such as type
, id
and name
. We can use these to locate (find) the elements.
Inputting text to form fields
We need to tell the robot to fill in the login form.
The robocorp.browser
library provides a page.fill()
function that can fill in text fields.
It takes two arguments: the selector
argument tells where to input and the value
argument tells what to input.
To be able to access the fill()
function, we need to first get the current page context.
This loads the current html page with all it's elements.
In our case, we can call the function like this to input maria
in the field that has the id
property with the value of username
.
To indicate to a selector that we are searching the id property, a
#
will be added before the value.
Let us run our robot to see how it works.
Filling and submitting the form
Now that we managed to input the username, we can do the same thing for the password.
Note: You should never write or commit your credentials in your robot code in a real project. Here we break that rule to keep things simple!
You can copy the password from the login form description (top-notch security right there!). Our log_in()
function looks like this now:
Submitting forms
As the last step, the robot needs to submit the form, which we can do by calling the page.click()
function.
It takes one argument, the selector
argument tells where to input.
We are going to identify the button by the text displayed on it Log in
.
Here's how the complete python code looks like now:
Run the robot one more time, and you should see it opening the browser, navigating to the intranet, and happily logging in, just like Maria does!
Optimizing your course completion time (time is money!)
You now have a general understanding of how robots are built (define tasks and functions, import libraries, run and iterate.). Congratulations!
You have real-world experience with typing out python code both in the editor.
You can now start taking shortcuts to save your precious time.
- Feel free to copy & paste the code blocks unmercifully. You can type things out if you want to.
- Do read the explanations.
- You will have plenty of chances to build robots from scratch and dive into details later.
- This course will not go away. You can always revisit it when you feel like it!
What we learned
- You can use the
page.fill()
function from therobocorp.browser
library to fill text input fields. - You can tell your robots which elements in a webpage to act on by writing locators (or selectors).
- You should not write or commit credentials directly into your project. We do it here to keep things simple, but you should never do this in the real world.
- Learning how to find and interact with elements is the bread and butter of web automation.