Collecting the results
All the sales data has been entered. Nice! After entering all the sales data, Maria takes a screenshot of the sales summary:
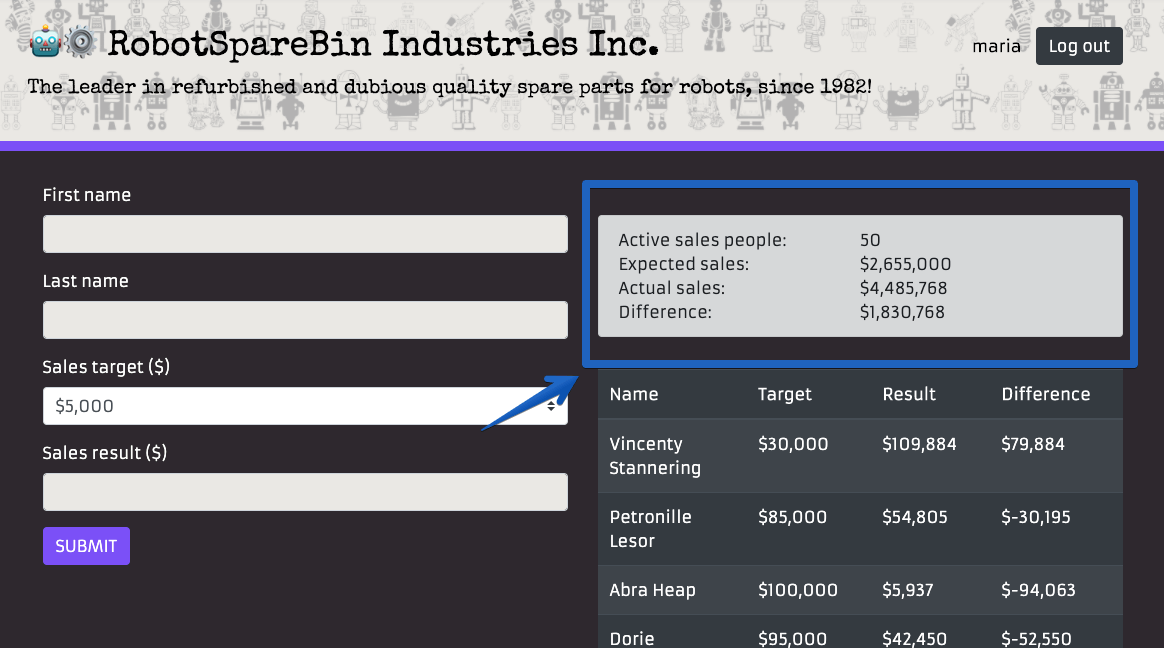
Our robot can handle this case too!
Let's add a new step to our task. We'll call it collect_results()
:
@task
def robot_spare_bin_python():
"""Insert the sales data for the week and export it as a PDF"""
browser.configure(
slowmo=100,
)
open_the_intranet_website()
log_in()
download_excel_file()
fill_form_with_excel_data()
collect_results()
We add a new function at the end of the file:
def collect_results():
"""Take a screenshot of the page"""
The robocorp.browser
module provides the page.screenshot()
function to help us with this step.
We give it a path where to save the image file.
This function will take a screenshot of the current view.
We will pass to page.screenshot()
the path to use for the screenshot image file.
We want the file to be called sales_summary.png
and added to the output
directory.
The output
folder is where Control Room will look for files generated by our robot. Check the robot.yaml
specification page for more information.
Our path for the file will be this: output/sales_summary.png
.
Our keyword implementation will be:
def collect_results():
"""Take a screenshot of the page"""
page = browser.page()
page.screenshot(path="output/sales_summary.png")
Our robot will now look like this:
from robocorp.tasks import task
from robocorp import browser
from RPA.HTTP import HTTP
from RPA.Excel.Files import Files
@task
def robot_spare_bin_python():
"""Insert the sales data for the week and export it as a PDF"""
browser.configure(
slowmo=100,
)
open_the_intranet_website()
log_in()
download_excel_file()
fill_form_with_excel_data()
collect_results()
def open_the_intranet_website():
"""Navigates to the given URL"""
browser.goto("https://robotsparebinindustries.com/")
def log_in():
"""Fills in the login form and clicks the 'Log in' button"""
page = browser.page()
page.fill("#username", "maria")
page.fill("#password", "thoushallnotpass")
page.click("button:text('Log in')")
def fill_and_submit_sales_form(sales_rep):
"""Fills in the sales data and click the 'Submit' button"""
page = browser.page()
page.fill("#firstname", sales_rep["First Name"])
page.fill("#lastname", sales_rep["Last Name"])
page.select_option("#salestarget", str(sales_rep["Sales Target"]))
page.fill("#salesresult", str(sales_rep["Sales"]))
page.click("text=Submit")
def download_excel_file():
"""Downloads excel file from the given URL"""
http = HTTP()
http.download(url="https://robotsparebinindustries.com/SalesData.xlsx", overwrite=True)
def fill_form_with_excel_data():
"""Read data from excel and fill in the sales form"""
excel = Files()
excel.open_workbook("SalesData.xlsx")
worksheet = excel.read_worksheet_as_table("data", header=True)
excel.close_workbook()
for row in worksheet:
fill_and_submit_sales_form(row)
def collect_results():
"""Take a screenshot of the page"""
page = browser.page()
page.screenshot(path="output/sales_summary.png")
We run the robot. A screenshot of the sales summary is stored in the output
folder at the end of the run.
Done!
- The
page.screenshot()
function of the robocorp.browser
module takes screenshots of the main view.
- The
output
folder is where we should put files that our robot generates.